Build and Deploy an MCP Server
Get started with MCP using a pre-built template with out-of-the-box deployment to Netlify
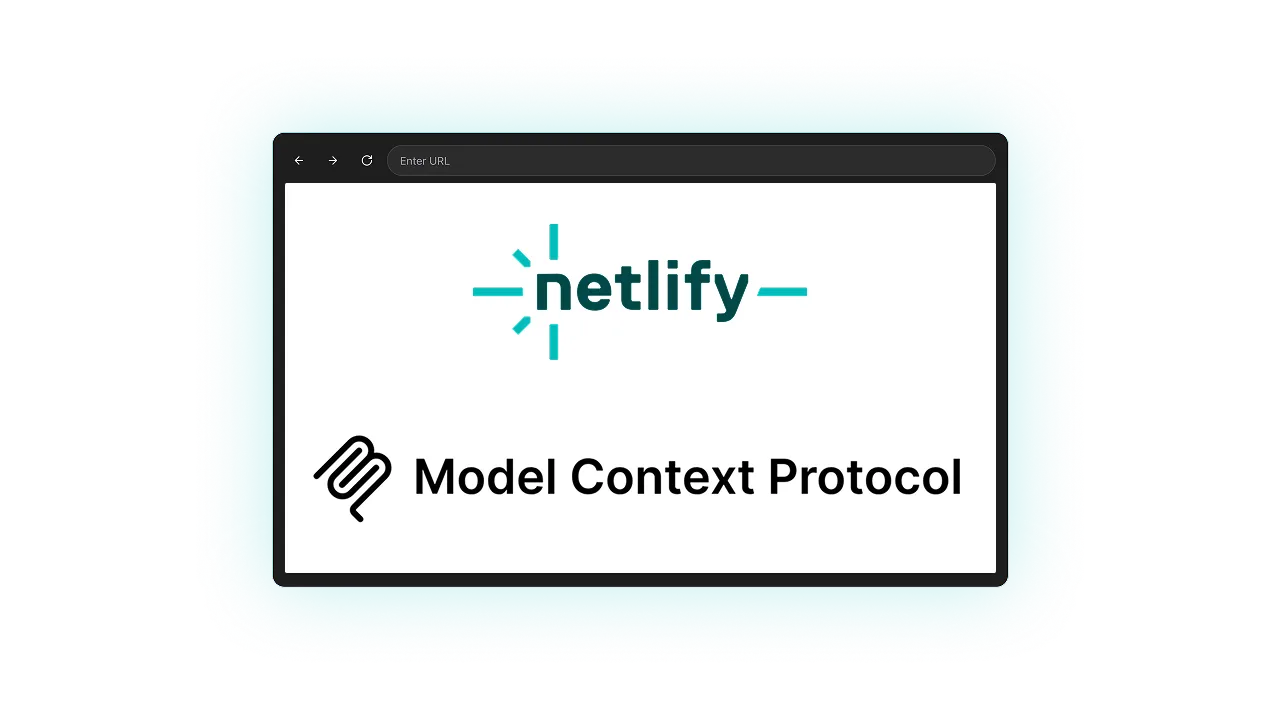
Barebones MCP Server for Netlify
This is a minimal implementation of a Model Context Protocol (MCP) server designed to be deployed to Netlify. The MCP server exposes tools and resources that can be used by AI assistants through the Model Context Protocol.
This project also includes a FastAPI client that provides a REST API for interacting with the MCP server, making it easy to test and integrate with other applications.
Features
MCP Server
- Simple serverless MCP server implementation
- Deployed on Netlify
- Includes a basic "run analysis report" tool
- Provides a resource with documentation on interpreting reports
MCP Client
- FastAPI REST API for interacting with the MCP server
- Interactive API documentation (Swagger UI)
- Easy testing and integration
- Python script for automated testing
Getting Started
Local Development
- Clone this repository
- Install dependencies:
npm install
- Start the development server:
npm run dev
The MCP server will be available locally at http://localhost:8888/mcp
Testing Your MCP Server
You can test your MCP server using either the MCP Inspector or directly with curl commands.
Using MCP Inspector
While the development server is running, you can test your MCP server using the MCP inspector:
npx @modelcontextprotocol/inspector npx mcp-remote@next http://localhost:8888/mcp
After deployment, you can test your deployed version:
npx @modelcontextprotocol/inspector npx mcp-remote@next https://your-site-name.netlify.app/mcp
Then open http://localhost:6274/ in your browser to interact with the MCP inspector.
Using curl
You can also test the MCP server directly using curl commands:
Initialize the MCP server:
curl -X POST http://localhost:8888/mcp \ -H "Content-Type: application/json" \ -d '{"jsonrpc":"2.0","method":"mcp/init","params":{},"id":"1"}'
List available tools:
curl -X POST http://localhost:8888/mcp \ -H "Content-Type: application/json" \ -d '{"jsonrpc":"2.0","method":"mcp/listTools","params":{},"id":"2"}'
Call a tool:
curl -X POST http://localhost:8888/mcp \ -H "Content-Type: application/json" \ -d '{"jsonrpc":"2.0","method":"mcp/callTool","params":{"name":"run-analysis-report","args":{"days":5}},"id":"3"}'
List available resources:
curl -X POST http://localhost:8888/mcp \ -H "Content-Type: application/json" \ -d '{"jsonrpc":"2.0","method":"mcp/listResources","params":{},"id":"4"}'
Read a resource:
curl -X POST http://localhost:8888/mcp \ -H "Content-Type: application/json" \ -d '{"jsonrpc":"2.0","method":"mcp/readResource","params":{"uri":"docs://interpreting-reports"},"id":"5"}'
Deployment
Deploying to Netlify
- Push this repository to GitHub
- Connect your repository to Netlify
- Configure the build settings:
- Build command: leave empty (no build required)
- Publish directory:
public
After deployment, your MCP server will be available at https://your-site-name.netlify.app/mcp
Using with Claude Desktop
To use this MCP server with Claude Desktop:
- Go to Claude Desktop settings
- Enable the MCP Server configuration
- Edit the configuration file:
{ "mcpServers": { "my-mcp": { "command": "npx", "args": [ "mcp-remote@next", "https://your-site-name.netlify.app/mcp" ] } } }
- Restart Claude Desktop
Using the MCP Client
The MCP client provides a REST API interface for interacting with the MCP server. It's built with FastAPI and offers a clean, modern API with automatic documentation.
Starting the Client
cd mcp-client
pip install -r requirements.txt
uvicorn main:app --reload
This will start the FastAPI server at http://localhost:8001. You can access the API documentation at http://localhost:8001/docs.
Managing the MCP Server and FastAPI Client
The template includes several scripts to manage both the MCP server and FastAPI client:
cd mcp-client
./start.sh # Start both services in the background
./stop.sh # Stop both services gracefully
./check_status.sh # Check if services are running and view logs
./test_client.py # Test the FastAPI client endpoints
These scripts ensure processes keep running in the background even after you close your terminal, properly manage log files, and provide clear status information.
Testing the Client
You can test the client using the provided test script:
cd mcp-client
./test_client.py
This will run a series of tests against the API endpoints and display the results.
API Endpoints
GET /server
- Get server informationGET /tools
- List available toolsPOST /tools/call
- Call a toolGET /resources
- List available resourcesPOST /resources/read
- Read a resource
For more details, refer to the MCP Client README.
Extending
Extending the MCP Server
You can extend this MCP server by adding more tools and resources to the getServer
function in netlify/functions/mcp-server.js
. Follow the existing examples and refer to the Model Context Protocol documentation for more information.
Extending the MCP Client
To add new endpoints to the MCP client, edit the main.py
file in the mcp-client
directory. The client is built with FastAPI, which makes it easy to add new routes and functionality.